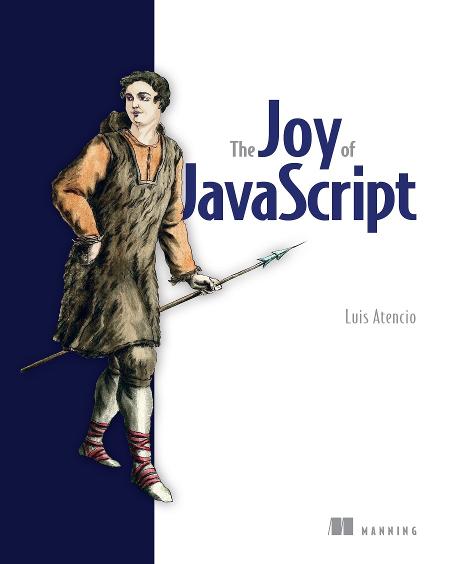
This chapter covers
- Refactoring imperative coding to a declarative, functional style
- Mastering JavaScript’s higher-order functions
- Introducing pure functions and immutability
- Combining pure logic with curry and composition
- Improving readability and structure of code with a point-free style
- Creating native function chains with the pipeline operator
If you want to see which features will be in mainstream programming languages tomorrow, then take a look at functional programming languages today
If objects are the fabric of JavaScript, functions represent the needles used to thread the pieces together. We can use functions in JavaScript to describe collections of objects (classes, constructor functions, and so on) and also to implement the business logic, the machinery that advances the state of our application. The reason why functions are so ubiquitous, versatile, and powerful in JavaScript is that they are objects too:
Function.prototype.__proto__.constructor === Object;
JavaScript has higher-order or first-class functions, which means you can pass them around as arguments to another function or as a return value. With higher-order functions, you can dramatically simplify complex patterns of software to a handful of functions, making JavaScript a lot more succinct than other mainstream languages such as Java and C#.